Feign调用者如何配置全局Header参数?
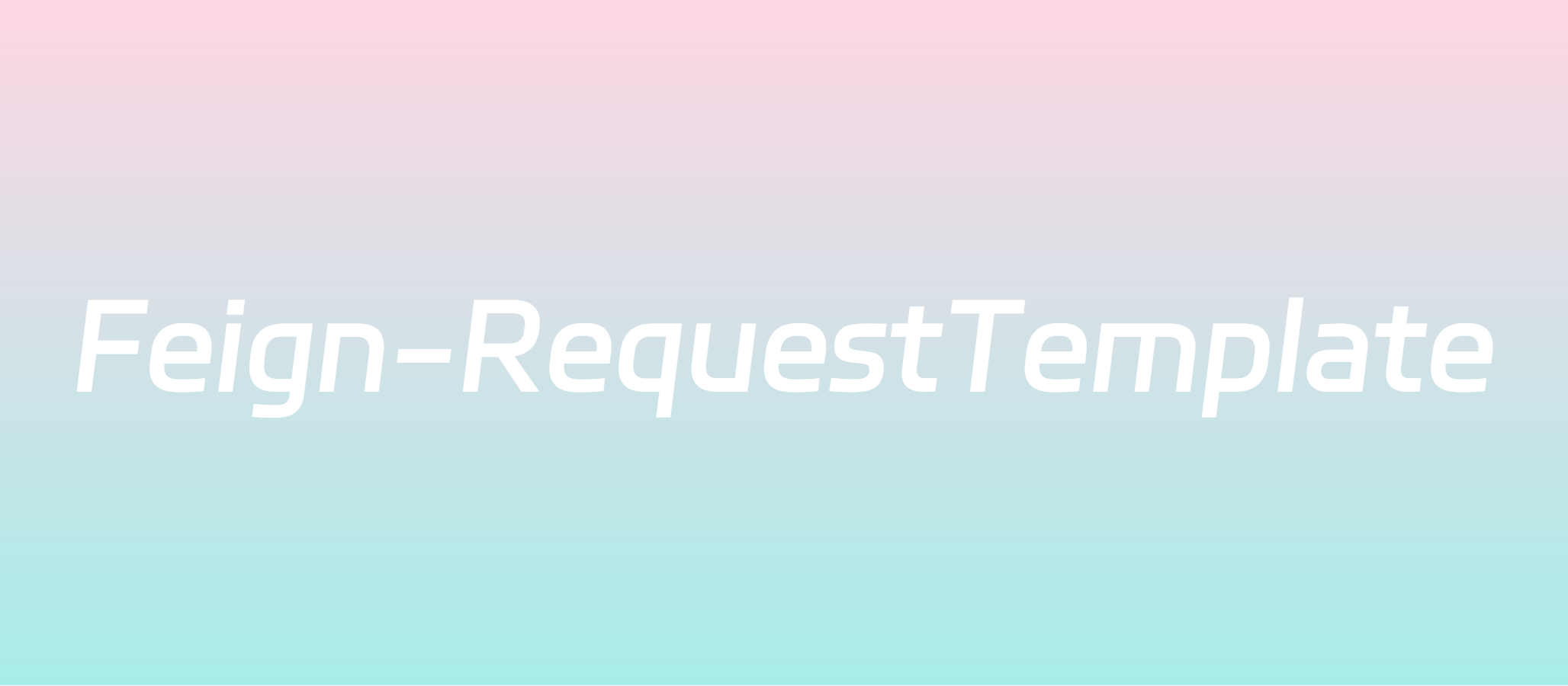
AI-摘要(由百度千帆大模型提供生成摘要能力)
Tianli GPT
AI初始化中...
介绍自己 🙈
生成本文简介 👋
推荐相关文章 📖
前往主页 🏠
前往爱发电购买
Feign调用者如何配置全局Header参数?
伴随作为Feign的调用者,如何配置一个全局的Header呢?
在我项目中的使用
1 | / |
RequestTemplate详细介绍
1. 路径和 URI 相关方法
-
设置请求的 URI 路径。
1
requestTemplate.uri("/new-path");
-
设置请求的方法(GET、POST、PUT 等)。
1
requestTemplate.method("POST");
-
添加查询参数(URL 参数)。
1
requestTemplate.query("key", "value1", "value2");
-
设置是否对 URL 中的斜杠进行解码。
1
requestTemplate.decodeSlash(false);
-
使用传入的参数解析模板中的占位符。占位符的形式一般是
{name}
。1
requestTemplate.resolve(new Object[]{"value"});
-
设置请求的目标 URL,通常是基础 URL。
1
requestTemplate.target("http://example.com");
2. 请求头相关方法
-
添加或设置请求头。
1
requestTemplate.header("Authorization", "Bearer token");
-
获取当前请求头的 Map 结构。
1
Map<String, Collection<String>> headers = requestTemplate.headers();
-
设置请求的字符集。
1
requestTemplate.requestCharset(StandardCharsets.UTF_8);
3. 请求体相关方法
-
直接设置请求体的内容和字符集。
1
requestTemplate.body("your request body".getBytes(), StandardCharsets.UTF_8);
-
以字符串形式设置请求体内容。
1
requestTemplate.body("your request body");
-
使用模板字符串设置请求体,可以包括占位符,在
resolve
时替换。1
requestTemplate.bodyTemplate("This is a body template with {param}");
4. 请求方法相关
-
获取与当前模板关联的MethodMetadata ,其中包含有关方法的元信息。
1
MethodMetadata metadata = requestTemplate.methodMetadata();
5. 克隆和复制
-
创建当前模板的副本。
1
RequestTemplate newTemplate = requestTemplate.clone();
-
使用另一个RequestTemplate 替换当前模板的内容。
1
requestTemplate.replace(newTemplate);
评论
匿名评论隐私政策